Scrollbar
Bootstrap 5 Scrollbar
Scrollbar method which allows you to create a custom scrollbar.
Note: Read the API tab to find all available options and advanced customization
Basic example
Scrollbar is initialized automatically when you add
data-mdb-perfect-scrollbar='true'
attribute to your container.
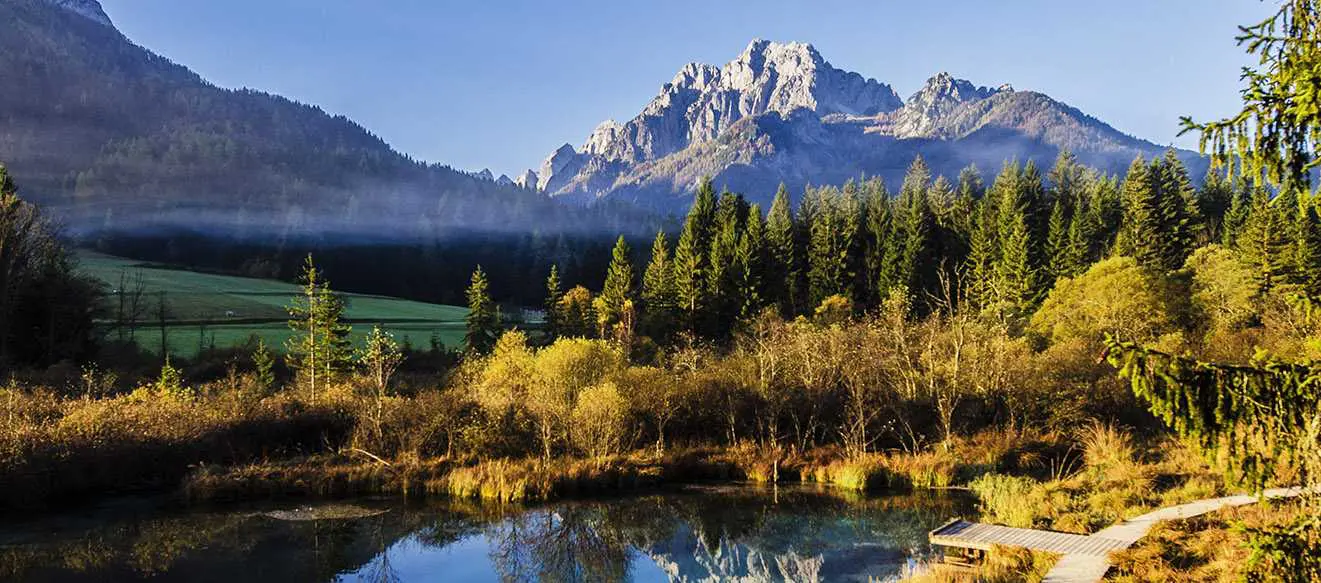
Options
JavaScript init
You can easily init scrollbar with JavaScript. You have to invoke
mdb.PerfectScrollbar()
and include options with in that.
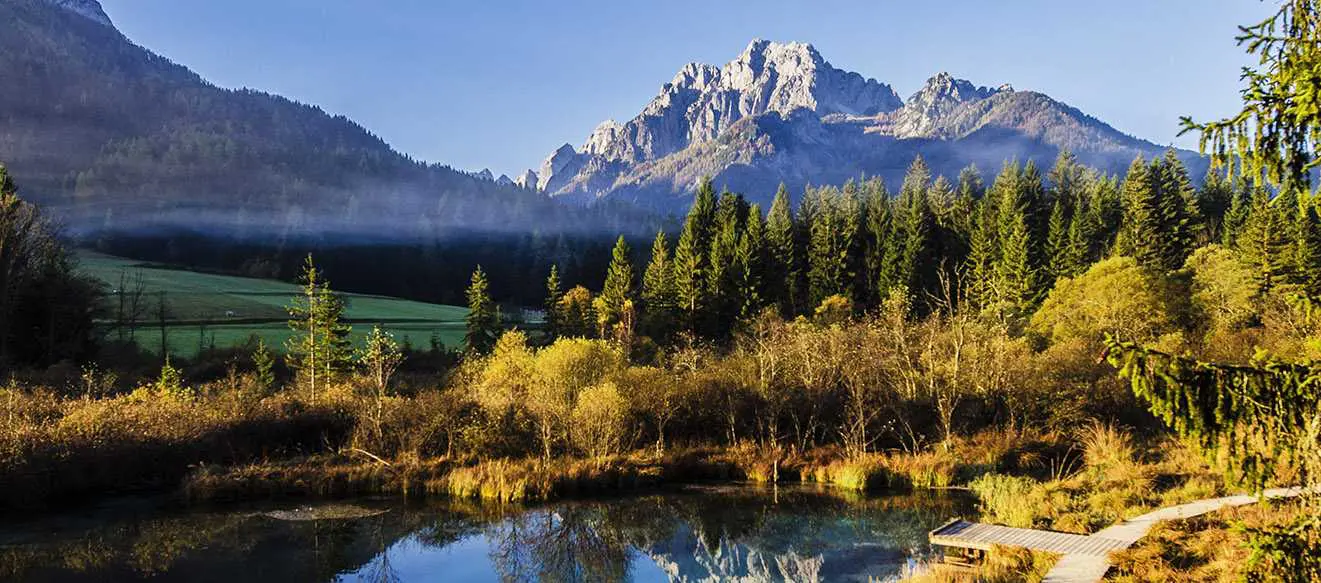
Data attributes
You can easily init scrollbar with options with data-mdb-attributes. You have to add
data-mdb-perefect-scrollbar
to your wrapper. If you want add options with
data-mdb-attr you have to add for example data-mdb-suppress-scroll-x='true'
to
your cointaier. .
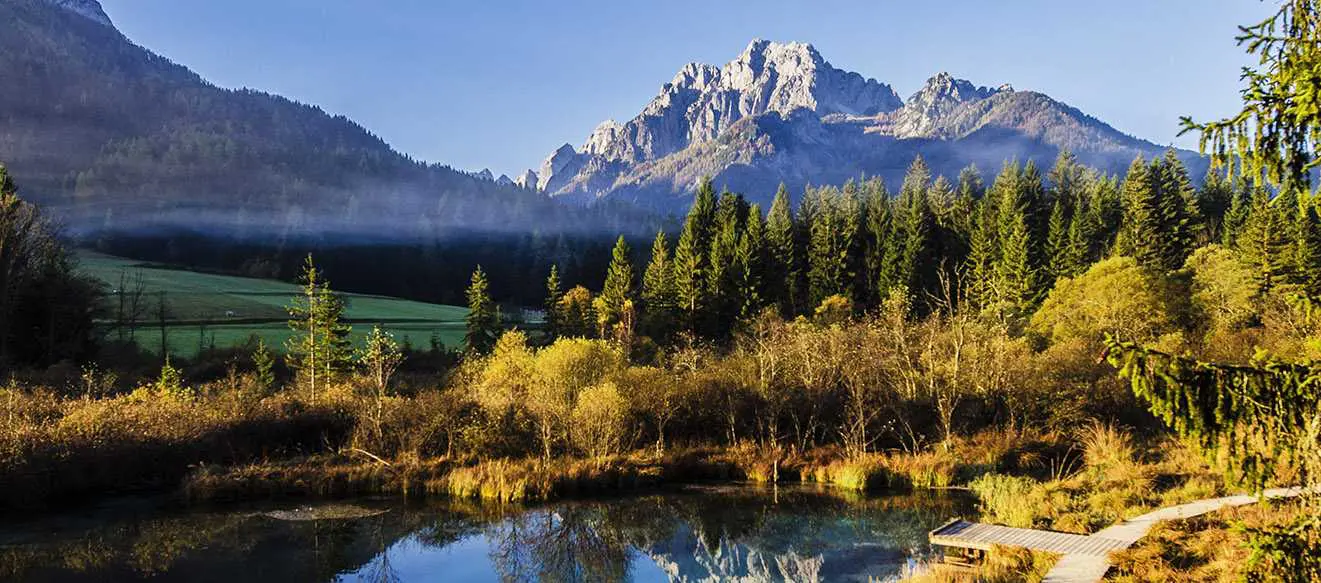
Colors example
Scrollbar's thumb and rail's colors can be customized with simple CSS.
Use class that describes the element of Scrollbar you would like to edit: .ps__rail-y
, .ps__rail-x
, .ps__thumb-y
, .ps__thumb-x
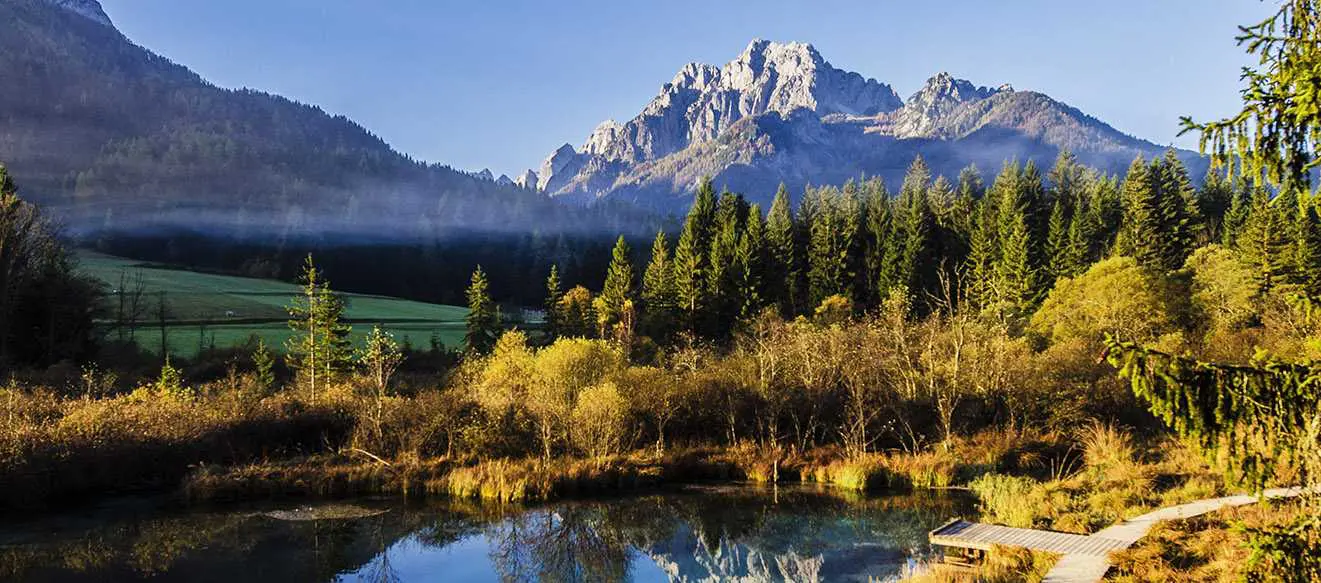
Events
PerfectScrollbar dispatches custom events.
- ScrollX
- ScrollY
- ScrollUp
- ScrollDown
- ScrollLeft
- ScrollRight
- ScrollXEnd
- ScrollYEnd
- ScrollXStart
- ScrollYStart
Example
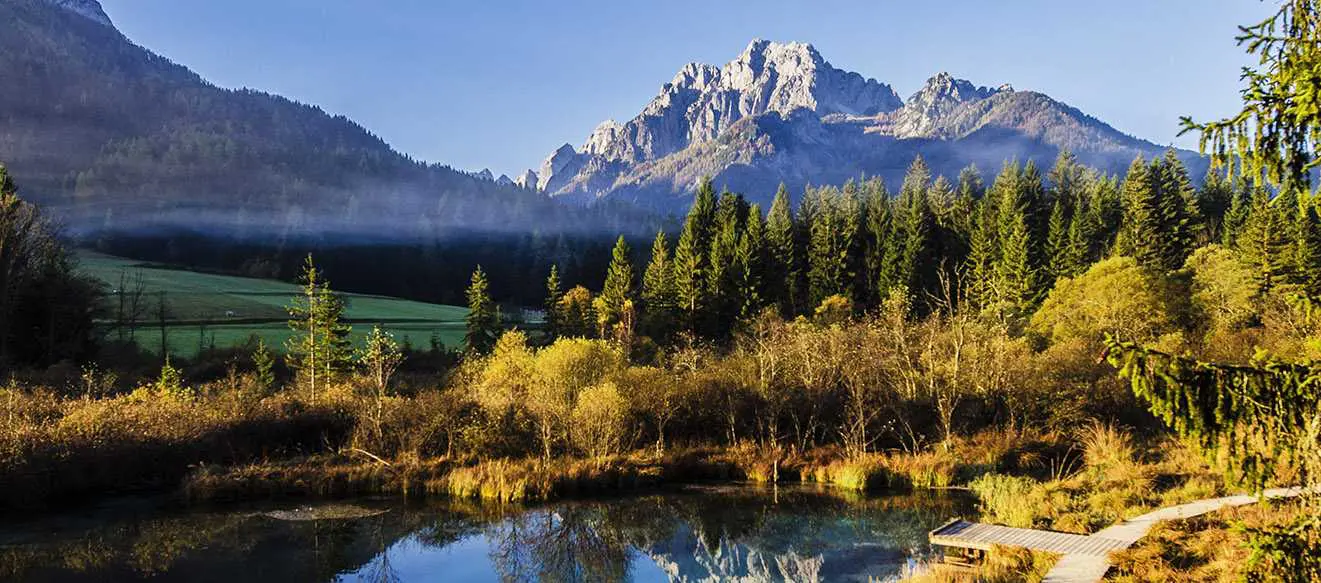
Usage
Via data attributes
<div data-mdb-perfect-scrollbar="true" data-mdb-handlers="click-rail"
style="position: relative; width: 600px; height: 400px;">
<img src="https://mdbootstrap.com/img/new/slides/041.webp" alt="..." style="height: 700px; width: 1000px;" />
</div>
Via JavaScript
var myPs = new mdb.PerfectScrollbar(document.getElementById('myPsID'), options)
Via jQuery
Note: By default, MDB does not include jQuery and you have to add it to the project on your own.
$('.example-class').perfectScrollbar(options);
Options
Name | Type | Default | Description |
---|---|---|---|
handlers
|
String[] |
['click-rail', 'drag-thumb', 'keyboard', 'wheel', 'touch']
|
It is a list of handlers to scroll the element. |
wheelSpeed
|
Number | 1 |
The scroll speed applied to mousewheel event. |
wheelPropagation
|
Boolean | true |
If this option is true, when the scroll reaches the end of the side, mousewheel event will be propagated to parent element. |
swipeEasing
|
Boolean | true |
If this option is true, swipe scrolling will be eased. |
minScrollbarLength
|
Boolean | null |
When set to an integer value, the thumb part of the scrollbar will not shrink below that number of pixels. |
maxScrollbarLength
|
Boolean | null |
When set to an integer value, the thumb part of the scrollbar will not expand over that number of pixels. |
scrollingThreshold
|
Number | 1000 |
This sets threshold for ps--scrolling-x and ps--scrolling-y classes to remain. In the default CSS, they make scrollbars shown regardless of hover state. The unit is millisecond. |
suppressScrollX
|
Boolean | false |
When set to true, the scrollbar in X-axis will not be available, regardless of the content width. |
suppressScrollY
|
Boolean | false |
When set to true, the scroll bar in Y-axis will not be available, regardless of the content height. |
scrollXMarginOffset
|
Number | 0 |
The number of pixels the content width can surpass the container width without enabling the X-axis scroll bar. Allows some "wiggle room" or "offset break", so that X-axis scroll bar is not enabled just because of a few pixels. |
scrollYMarginOffset
|
Number | 0 |
The number of pixels the content width can surpass the container width without enabling the X-axis scroll bar. Allows some "wiggle room" or "offset break", so that X-axis scroll bar is not enabled just because of a few pixels. |
Methods
Name | Description | Example |
---|---|---|
dispose
|
Destroy ps | myPs.dispose() |
getInstance
|
Static method which allows you to get the perfect scrollbar instance associated to a DOM element. |
PerfectScrollbar.getInstance(myPs)
|
update |
Update ps | myPs.update() |
var myPs = document.getElementById('myPsID')
var ps = new mdb.PerfectScrollbar(myPs)
ps.dispose()
Events
Name | Description |
---|---|
scrollX.mdb.ps
|
This event fires when the x-axis is scrolled in either direction. |
var myPs = document.getElementById('element-id')
myPs.addEventListener('scrollX.mdb.ps', (e) => {
// do something...
})
Name | Description |
---|---|
scrollY.mdb.ps
|
This event fires when the y-axis is scrolled in either direction. |
var myPs = document.getElementById('element-id')
myPs.addEventListener('scrollY.mdb.ps', (e) => {
// do something...
})
Name | Description |
---|---|
scrollUp.mdb.ps
|
This event fires when scrolling upwards. |
var myPs = document.getElementById('element-id')
myPs.addEventListener('scrollUp.mdb.ps', (e) => {
// do something...
})
Name | Description |
---|---|
scrollDown.mdb.ps
|
This event fires when scrolling downwards. |
var myPs = document.getElementById('element-id')
myPs.addEventListener('scrollDown.mdb.ps', (e) => {
// do something...
})
Name | Description |
---|---|
scrollLeft.mdb.ps
|
This event fires when scrolling to the left. |
var myPs = document.getElementById('element-id')
myPs.addEventListener('scrollRight.mdb.ps', (e) => {
// do something...
})
Name | Description |
---|---|
scrollRight.mdb.ps
|
This event fires when scrolling to the right. |
var myPs = document.getElementById('element-id')
myPs.addEventListener('scrollLeft.mdb.ps', (e) => {
// do something...
})
Name | Description |
---|---|
scrollLeft.mdb.ps
|
This event fires when scrolling to the left. |
var myPs = document.getElementById('element-id')
myPs.addEventListener('scrollRight.mdb.ps', (e) => {
// do something...
})
Name | Description |
---|---|
scrollYStart.mdb.ps
|
This event fires when scrolling to the right. |
var myPs = document.getElementById('element-id')
myPs.addEventListener('scrollLeft.mdb.ps', (e) => {
// do something...
})
Name | Description |
---|---|
scrollYStart.mdb.ps
|
This event fires when scrolling reaches the start of the y-axis. |
var myPs = document.getElementById('element-id')
myPs.addEventListener('scrollYStart.mdb.ps', (e) => {
// do something...
})
Name | Description |
---|---|
scrollXStart.mdb.ps
|
This event fires when scrolling reaches the start of the x-axis. |
var myPs = document.getElementById('element-id')
myPs.addEventListener('scrollXStart.mdb.ps', (e) => {
// do something...
})
Name | Description |
---|---|
scrollXEnd.mdb.ps
|
This event fires when scrolling reaches the end of the x-axis. |
var myPs = document.getElementById('element-id')
myPs.addEventListener('scrollXEnd.mdb.ps', (e) => {
// do something...
})
Name | Description |
---|---|
scrollYEnd.mdb.ps
|
This event fires when scrolling reaches the end of the y-axis (useful for infinite scroll). |
var myPs = document.getElementById('element-id')
myPs.addEventListener('scrollYEnd.mdb.ps', (e) => {
// do something...
})
Import
MDB UI KIT also works with module bundlers. Use the following code to import this component:
import { PerfectScrollbar } from 'mdb-ui-kit';