React Bootstrap Cards
React Cards - Bootstrap 4 & Material Design
Note: This documentation is for an older version of Bootstrap (v.4). A
newer version is available for Bootstrap 5. We recommend migrating to the latest version of our product - Material Design for
Bootstrap 5.
Go to docs v.5
Bootstrap cards are components which display content build of different elements with characteristic shadows, depth and hover effects.
It is hard to think of a better way of displaying your content to users other than by cards. Some of the biggest players like Facebook or Google are well aware of that, as you might have noticed in almost all their products.
Cards provide you with clarity, clean content categorisation and attractive form of presenting it to the users.
MDB enhances Bootstrap cards with characteristic Material Design features, such as slight shadows, depth, cascading or waves effects. Apart from that, it enriches it with additional optional animations, social icons, avatars and various other effects not available in a native Bootstrap.
Basic example
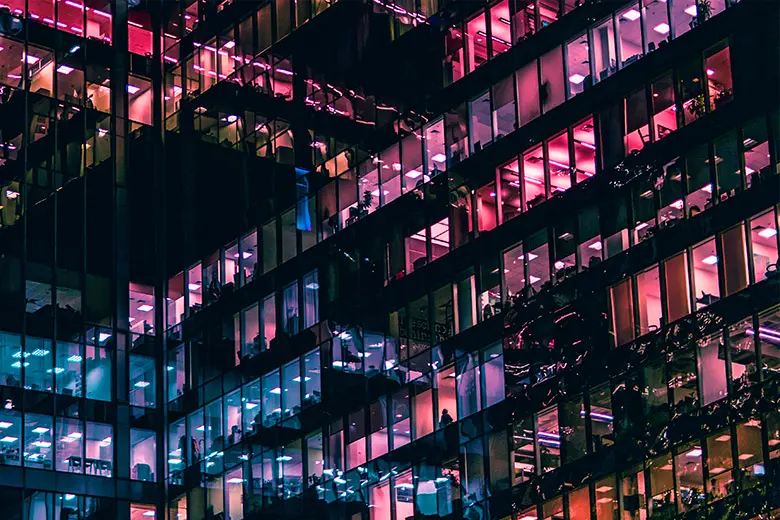
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Button
import React from 'react';
import { MDBBtn, MDBCard, MDBCardBody, MDBCardImage, MDBCardTitle, MDBCardText, MDBCol } from 'mdbreact';
const CardExample = () => {
return (
<MDBCol>
<MDBCard style={{ width: "22rem" }}>
<MDBCardImage className="img-fluid" src="https://mdbootstrap.com/img/Photos/Others/images/43.webp" waves />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make
up the bulk of the card's content.
</MDBCardText>
<MDBBtn href="#">MDBBtn</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
)
}
export default CardExample;
Waves effect
.webp)
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Button
import React from 'react';
import { MDBBtn, MDBCard, MDBCardBody, MDBCardImage, MDBCardTitle, MDBCardText, MDBCol } from 'mdbreact';
const CardExample = () => {
return (
<MDBCol style={{ maxWidth: "22rem" }}>
<MDBCard>
<MDBCardImage className="img-fluid" src="https://mdbootstrap.com/img/Mockups/Lightbox/Thumbnail/img%20(67).webp"
waves />
<MDBCardBody>
<MDBCardTitle>Card title</MDBCardTitle>
<MDBCardText>Some quick example text to build on the card title and make up the bulk of the card's content.</MDBCardText>
<MDBBtn href="#">Click</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
)
}
export default CardExample;
Cascading cards MDB Pro component
Wider
Narrower
Culinary
Cheat day inspirations
Ut enim ad minima veniam, quis nostrum exercitationem ullam corporis suscipit laboriosam, nisi ut aliquid ex ea commodi.
Button
import React from 'react';
import { MDBBtn, MDBCard, MDBCardBody, MDBCardImage, MDBCardTitle, MDBCardText, MDBRow, MDBCol, MDBView, MDBIcon } from 'mdbreact';
const CardExample = () => {
return (
<MDBRow>
<MDBCol md='4'>
<MDBCard wide cascade>
<MDBView cascade>
<MDBCardImage
hover
overlay='white-slight'
className='card-img-top'
src='https://mdbcdn.b-cdn.net/img/Photos/Horizontal/People/6-col/img%20%283%29.webp'
alt='Card cap'
/>
</MDBView>
<MDBCardBody cascade className='text-center'>
<MDBCardTitle className='card-title'>
<strong>Alice Mayer</strong>
</MDBCardTitle>
<p className='font-weight-bold blue-text'>Photographer</p>
<MDBCardText>
Sed ut perspiciatis unde omnis iste natus sit voluptatem
accusantium doloremque laudantium, totam rem aperiam.{' '}
</MDBCardText>
<MDBCol md='12' className='d-flex justify-content-center'>
<a href='!#' className='px-2 fa-lg li-ic'>
<MDBIcon fab icon='linkedin-in'></MDBIcon>
</a>
<a href='!#' className='px-2 fa-lg tw-ic'>
<MDBIcon fab icon='twitter'></MDBIcon>
</a>
<a href='!#' className='px-2 fa-lg fb-ic'>
<MDBIcon fab icon='facebook-f'></MDBIcon>
</a>
</MDBCol>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md='4'>
<MDBCard narrow>
<MDBView cascade>
<MDBCardImage
hover
overlay='white-slight'
className='card-img-top'
src='https://mdbootstrap.com/img/Photos/Lightbox/Thumbnail/img%20(147).webp'
alt='food'
/>
</MDBView>
<MDBCardBody>
<h5 className='pink-text'>
<MDBIcon icon='utensils' /> Culinary
</h5>
<MDBCardTitle className='font-weight-bold'>
Cheat day inspirations
</MDBCardTitle>
<MDBCardText>
Sed ut perspiciatis unde omnis iste natus sit voluptatem
accusantium doloremque laudantium, totam rem aperiam.
</MDBCardText>
<MDBBtn color='unique'>Button</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md='4'>
<MDBCard>
<MDBCardImage
hover
overlay='white-light'
className='card-img-top'
src='https://mdbcdn.b-cdn.net/img/Photos/Others/men.webp'
alt='man'
/>
<MDBCardBody cascade className='text-center'>
<MDBCardTitle className='card-title'>
<strong>Billy Coleman</strong>
</MDBCardTitle>
<p className='font-weight-bold blue-text'>Wev developer</p>
<MDBCardText>
Sed ut perspiciatis unde omnis iste natus sit voluptatem
accusantium doloremque laudantium, totam rem aperiam.{' '}
</MDBCardText>
<MDBCol md='12' className='d-flex justify-content-center'>
<MDBBtn rounded floating color='fb'>
<MDBIcon size='lg' fab icon='facebook-f'></MDBIcon>
</MDBBtn>
<MDBBtn rounded floating color='tw'>
<MDBIcon size='lg' fab icon='twitter'></MDBIcon>
</MDBBtn>
<MDBBtn rounded floating color='dribbble'>
<MDBIcon size='lg' fab icon='dribbble'></MDBIcon>
</MDBBtn>
</MDBCol>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
)
}
export default CardExample;
Cascading panels MDB Pro component
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam dignissimos neque rem nihil ratione est placeat vel, natus non quos laudantium veritatis sequi.Ut enim ad minima veniam, quis nostrum exercitationem ullam corporis suscipit laboriosam, nisi ut aliquid ex ea commodi.
Title of the news
26.07.2017
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam dignissimos neque rem nihil ratione est placeat vel, natus non quos laudantium veritatis sequi.Ut enim ad minima veniam, quis nostrum.
Read more
Marta
Deserve for her own card
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam dignissimos neque rem nihil ratione est placeat vel, natus non quos laudantium veritatis sequi.Ut enim ad minima veniam, quis nostrum exercitationem ullam corporis suscipit laboriosam.
import React from 'react';
import { MDBBtn, MDBCard, MDBCardBody, MDBCardImage, MDBCardTitle, MDBCardText, MDBRow, MDBCol, MDBIcon } from
'mdbreact';
const CardExample = () => {
return (
<MDBRow>
<MDBCol col='4'>
<MDBCard narrow>
<MDBCardImage
className='view view-cascade gradient-card-header purple-gradient'
cascade
tag='div'
>
<h2 className='h2-responsive'>Mattonit</h2>
<p>The Boar</p>
<div className='text-center'>
<MDBBtn color='purple' floating size='sm'>
<MDBIcon fab icon='facebook-f' size="lg"/>
</MDBBtn>
<MDBBtn color='purple' floating size='sm'>
<MDBIcon fab icon='twitter' size="lg"/>
</MDBBtn>
<MDBBtn color='purple' floating size='sm'>
<MDBIcon fab icon='google-plus-g' size="lg"/>
</MDBBtn>
</div>
</MDBCardImage>
<MDBCardBody cascade className='text-center'>
<MDBCardText>
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam
dignissimos neque rem nihil ratione est placeat vel, natus non
quos laudantium veritatis sequi.Ut enim ad minima veniam, quis
nostrum exercitationem ullam corporis suscipit laboriosam, nisi ut
aliquid ex ea commodi.
</MDBCardText>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol col='4'>
<MDBCard wide>
<MDBCardImage
className='view view-cascade gradient-card-header peach-gradient'
cascade
tag='div'
>
<h2 className='h2-responsive mb-2'>Title of the news</h2>
<p>
<MDBIcon icon='calendar-alt' /> 26.07.2017
</p>
</MDBCardImage>
<MDBCardBody cascade className='text-center'>
<MDBCardText>
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam
dignissimos neque rem nihil ratione est placeat vel, natus non
quos laudantium veritatis sequi.Ut enim ad minima veniam, quis
nostrum.
</MDBCardText>
<a
href='!#'
className='orange-text mt-1 d-flex justify-content-end align-items-center'
>
<h5 className=''>
Read more{' '}
<MDBIcon
icon='chevron-right'
className='ml-2'
size='sm'
></MDBIcon>
</h5>
</a>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol col='4'>
<MDBCard>
<MDBCardImage
className='blue-gradient white-text d-flex justify-content-center align-items-center flex-column p-4 rounded'
tag='div'
>
<h2>Marta</h2>
<p>Deserves her own card</p>
</MDBCardImage>
<MDBCardBody cascade className='text-center'>
<MDBCardText>
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Voluptatibus, ex, recusandae. Facere modi sunt, quod quibusdam
dignissimos neque rem nihil ratione est placeat vel, natus non
quos laudantium veritatis sequi.Ut enim ad minima veniam, quis
nostrum.
</MDBCardText>
<hr />
<div className='text-center'>
<MDBIcon fab icon='twitter' className='tw-ic mr-3' size='lg' />
<MDBIcon
fab
icon='linkedin-in'
className='li-ic my-3'
size='lg'
/>
<MDBIcon fab icon='facebook-f' className='fb-ic ml-3' size='lg' />
</div>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
)
}
export default CardExample;
Reversed cascade MDB Pro component
import React from 'react';
import { MDBCard, MDBCardBody, MDBCardImage, MDBCardTitle, MDBCardText, MDBRow, MDBCol, MDBIcon } from 'mdbreact';
const CardExample = () => {
return (
<MDBRow>
<MDBCol style={{ maxWidth: "40rem" }}>
<MDBCard reverse>
<MDBCardImage cascade style={{ height: '20rem' }} src="https://mdbootstrap.com/img/Photos/Slides/img%20(70).webp" />
<MDBCardBody cascade className="text-center">
<MDBCardTitle>My adventure</MDBCardTitle>
<h5 className="indigo-text"><strong>Photography</strong></h5>
<MDBCardText>Sed ut perspiciatis unde omnis iste natus sit voluptatem accusantium doloremque laudantium, totam
rem aperiam.</MDBCardText>
<a href="#!" className="icons-sm li-ic ml-1">
<MDBIcon fab icon="linkedin-in" /></a>
<a href="#!" className="icons-sm tw-ic ml-1">
<MDBIcon fab icon="twitter" /></a>
<a href="#!" className="icons-sm fb-ic ml-1">
<MDBIcon fab icon="facebook-f" /></a>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
)
}
export default CardExample;
Dark version MDB Pro component
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Read more
import React from 'react';
import { MDBCard, MDBCardBody, MDBCardImage, MDBCardTitle, MDBCardText, MDBRow, MDBCol, MDBIcon } from 'mdbreact';
const CardExample = () => {
return (
<MDBRow>
<MDBCol md='4'>
<MDBCard>
<MDBCardImage
top
src='https://mdbcdn.b-cdn.net/img/Photos/Horizontal/Work/4-col/img%20%2821%29.webp'
overlay='white-slight'
hover
waves
alt='MDBCard image cap'
/>
<MDBCardBody className='elegant-color white-text rounded-bottom'>
<a href='#!' className='activator waves-effect waves-light mr-4'>
<MDBIcon icon='share-alt' className='white-text' />
</a>
<MDBCardTitle>MDBCard Title</MDBCardTitle>
<hr className='hr-light' />
<MDBCardText className='white-text'>
Some quick example text to build on the card title and make up the
bulk of the card's content.
</MDBCardText>
<a href='#!' className='black-text d-flex justify-content-end'>
<h5 className='white-text'>
Read more
<MDBIcon icon='angle-double-right' className='ml-2' />
</h5>
</a>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
)
}
export default CardExample;
Testimonial card MDB Pro component
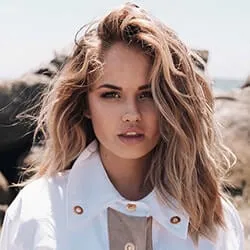
Anna Doe
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Eos, adipisci
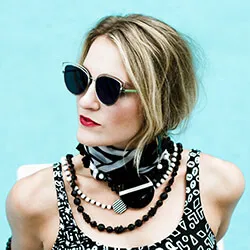
Martha Smith
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Eos, adipisci
import React from 'react';
import { MDBCard, MDBCardBody, MDBCardUp, MDBAvatar, MDBRow, MDBCol, MDBIcon } from 'mdbreact';
const CardExample = () => {
return (
<MDBRow>
<MDBCol md='4'>
<MDBCard testimonial>
<MDBCardUp className='indigo lighten-1' />
<MDBAvatar className='mx-auto white'>
<img
src='https://mdbcdn.b-cdn.net/img/Photos/Avatars/img%20%2810%29.webp'
alt=''
/>
</MDBAvatar>
<MDBCardBody>
<h4 className='card-title'>Anna Doe</h4>
<hr />
<p>
<MDBIcon icon='quote-left' /> Lorem ipsum dolor sit amet,
consectetur adipisicing elit. Eos, adipisci{' '}
<MDBIcon icon='quote-right' />
</p>
</MDBCardBody>
</MDBCard>
</MDBCol>
<MDBCol md='4'>
<MDBCard testimonial>
<MDBCardUp gradient='aqua' />
<MDBAvatar className='mx-auto white'>
<img
src='https://mdbcdn.b-cdn.net/img/Photos/Avatars/img%20%2831%29.webp'
alt=''
/>
</MDBAvatar>
<MDBCardBody>
<h4 className='card-title'>Martha Smith</h4>
<hr />
<p>
<MDBIcon icon='quote-left' /> Lorem ipsum dolor sit amet,
consectetur adipisicing elit. Eos, adipisci{' '}
<MDBIcon icon='quote-right' />
</p>
</MDBCardBody>
</MDBCard>
</MDBCol>
</MDBRow>
)
}
export default CardExample;
Image overlay MDB Pro component
Marketing
This is card title
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Repellat fugiat, laboriosam, voluptatem, optio vero odio nam sit officia accusamus minus error nisi architecto nulla ipsum dignissimos. Odit sed qui, dolorum!
View projectSoftware
This is card title
Lorem ipsum dolor sit amet, consectetur adipisicing elit. Repellat fugiat, laboriosam, voluptatem, optio vero odio nam sit officia accusamus minus error nisi architecto nulla ipsum dignissimos. Odit sed qui, dolorum!
View project
import React from 'react';
import { MDBCard, MDBCardTitle, MDBBtn, MDBRow, MDBCol, MDBIcon } from 'mdbreact';
const CardExample = () => {
return (
<MDBRow>
<MDBCol md='4'>
<MDBCard
className='card-image'
style={{
backgroundImage:
"url('https://mdbcdn.b-cdn.net/img/Photos/Horizontal/Work/4-col/img%20%2814%29.webp')"
}}
>
<div className='text-white text-center d-flex align-items-center rgba-black-strong py-5 px-4'>
<div>
<h5 className='pink-text'>
<MDBIcon icon='chart-pie' /> Marketing
</h5>
<MDBCardTitle tag='h3' className='pt-2'>
<strong>This is card title</strong>
</MDBCardTitle>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Repellat fugiat, laboriosam, voluptatem, optio vero odio nam sit
officia accusamus minus error nisi architecto nulla ipsum
dignissimos. Odit sed qui, dolorum!
</p>
<MDBBtn color='pink'>
<MDBIcon icon='clone left' /> View project
</MDBBtn>
</div>
</div>
</MDBCard>
</MDBCol>
<MDBCol md='4'>
<MDBCard
className='card-image'
style={{
backgroundImage:
"url('https://mdbootstrap.com/img/Photos/Horizontal/City/6-col/img%20(47).webp')"
}}
>
<div className='text-white text-center d-flex align-items-center rgba-indigo-strong py-5 px-4'>
<div>
<h5 className='orange-text'>
<MDBIcon icon='desktop' /> Software
</h5>
<MDBCardTitle tag='h3' className='pt-2'>
<strong>This is card title</strong>
</MDBCardTitle>
<p>
Lorem ipsum dolor sit amet, consectetur adipisicing elit.
Repellat fugiat, laboriosam, voluptatem, optio vero odio nam sit
officia accusamus minus error nisi architecto nulla ipsum
dignissimos. Odit sed qui, dolorum!
</p>
<MDBBtn color='deep-orange'>
<MDBIcon icon='clone left' /> View project
</MDBBtn>
</div>
</div>
</MDBCard>
</MDBCol>
</MDBRow>
)
}
export default CardExample;
Card groups
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
import React from "react";
import { MDBCard, MDBCardTitle, MDBBtn, MDBCardGroup, MDBCardImage, MDBCardText, MDBCardBody } from "mdbreact";
const CardExample = () => {
return (
<MDBCardGroup>
<MDBCard>
<MDBCardImage src="https://mdbootstrap.com/img/Photos/Others/images/49.webp" alt="MDBCard image cap" top hover
overlay="white-slight" />
<MDBCardBody>
<MDBCardTitle tag="h5">Panel title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up
the bulk of the card's content.
</MDBCardText>
<MDBBtn color="primary" size="md">
read more
</MDBBtn>
</MDBCardBody>
</MDBCard>
<MDBCard>
<MDBCardImage src="https://mdbootstrap.com/img/Photos/Others/images/48.webp" alt="MDBCard image cap" top hover
overlay="white-slight" />
<MDBCardBody>
<MDBCardTitle tag="h5">Panel title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up
the bulk of the card's content.
</MDBCardText>
<MDBBtn color="primary" size="md">
read more
</MDBBtn>
</MDBCardBody>
</MDBCard>
<MDBCard>
<MDBCardImage src="https://mdbootstrap.com/img/Photos/Others/images/77.webp" alt="MDBCard image cap" top hover
overlay="white-slight" />
<MDBCardBody>
<MDBCardTitle tag="h5">Panel title</MDBCardTitle>
<MDBCardText>
Some quick example text to build on the card title and make up
the bulk of the card's content.
</MDBCardText>
<MDBBtn color="primary" size="md">
read more
</MDBBtn>
</MDBCardBody>
</MDBCard>
</MDBCardGroup>
);
}
export default CardExample;
React Cards - API
In this section you will find advanced information about the Card component. You will find out which modules are required, what are the possibilities of configuring the component, and what events and methods you can use in working with it.
Cards import statement
In order to use Cards make sure you have imported proper modules first.
import { MDBCard, MDBCardBody, MDBCardImage, MDBCardUp, MDBCardTitle, MDBCardText, MDBAvatar, MDBFlippingCard } from "mdbreact";
API Reference: Card Properties
The table below shows the configuration options of the MDBCard component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
border |
String |
|
Sets border color | <MDBCard border="default" /> |
cascade |
Boolean | false |
Switches the Card to cascade style mode | <MDBCard cascade /> |
className |
String |
|
Adds custom classes | <MDBCard className="customClass" /> |
collection |
Boolean | false |
Switches on styles for collection Card | <MDBCard collection /> |
ecommerce |
Boolean | false |
Switches on styles for ecommerce Card | <MDBCard ecommerce /> |
narrow |
Boolean | false |
Makes cascading Card content body wider than header | <MDBCard narrow /> |
news |
Boolean | false |
Switches on styles for news Card | <MDBCard news /> |
personal |
Boolean | false |
Switches on styles for personal Card | <MDBCard personal /> |
pricing |
Boolean | false |
Switches on styles for pricing Card | <MDBCard pricing /> |
reverse |
Boolean | false |
Makes Card header wider and places content body on the header | <MDBCard reverse /> |
tag |
String | div |
Changes the default html tag for Card component | <MDBCard tag="div" /> |
testimonial |
Boolean | false |
Switches on styles for testimonial Card | <MDBCard testimonial /> |
wide |
Boolean | false |
Makes cascading Card header wider than content body | <MDBCard wide /> |
API Reference: CardBody Properties
The table below shows the configuration options of the MDBCardBody component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
cascade |
Boolean | false |
Switches the CardBody to cascade style mode | <MDBCardBody cascade /> |
className |
String |
|
Adds custom classes | <MDBCardBody className="customClass" /> |
tag |
String | div |
Changes the default html tag for CardBody component | <MDBCardBody tag="div" /> |
API Reference: CardImage Properties
The table below shows the configuration options of the MDBCardImage component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
alt |
String |
|
Alternative text when image does not load | <MDBCardImage alt="Image" /> |
cascade |
Boolean | false |
Switches the CardImage to cascade style mode | <MDBCardImage cascade /> |
className |
String |
|
Adds custom classes | <MDBCardImage className="customClass" /> |
hover |
Boolean | false |
Switches on hover behaviour | <MDBCardImage hover /> |
overlay |
String | white-slight |
Sets the color of hover overlay | <MDBCardImage overlay="white-light" /> |
src |
String |
|
URL of the image | <MDBCardImage src="https://yourimage" /> |
tag |
String | img |
Changes the default html tag for CardImage component | <MDBCardImage tag="div" /> |
top |
Boolean | false |
Makes image fluid | <MDBCardImage top /> |
waves |
Boolean | true |
Switches on waves effect | <MDBCardImage waves /> |
API Reference: CardUp Properties
The table below shows the configuration options of the MDBCardUp component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
className |
String |
|
Adds custom classes | <MDBCardUp className="customClass" /> |
color |
String |
|
Sets background color | <MDBCardUp color="indigo" /> |
gradient |
String |
|
Sets background gradient | <MDBCardUp gradient="aqua" /> |
tag |
String | img |
Changes the default html tag for CardUp component | <MDBCardUp tag="div" /> |
API Reference: CardTitle Properties
The table below shows the configuration options of the MDBCardTitle component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
className |
String |
|
Adds custom classes | <MDBCardTitle className="customClass" /> |
sub |
Boolean | false |
Styles title as subtitle | <MDBCardTitle sub /> |
tag |
String | img |
Changes the default html tag for component | <MDBCardTitle tag="div" /> |
API Reference: CardText Properties
The table below shows the configuration options of the MDBCardText component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
className |
String |
|
Adds custom classes | <MDBCardText className="customClass" /> |
muted |
Boolean | false |
Mutes the text color | <MDBCardText muted /> |
small |
Boolean | false |
Uses html small as component's tag |
<MDBCardText small /> |
tag |
String | p |
Changes the default html tag for component | <MDBCardText tag="div" /> |
API Reference: CardVideo Properties
The table below shows the configuration options of the MDBCardVideo component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
allowFullScreen |
boolean |
|
If set, applies the allowFullScreen param | <MDBCardVideo allowFullScreen ... /> |
className |
string |
|
Adds custom class to the element | <MDBCardVideo className="myClass" ... /> |
height |
number |
|
Defines component height | <MDBCardVideo height={300} ... /> |
id |
string |
|
Adds id to the element | <MDBCardVideo id="myId" ... /> |
name |
string |
|
Adds name attribute | <MDBCardVideo name="myMDBCardVideo" ... /> |
onMouseOver |
function |
|
Function fired on onMouseOver event | <MDBCardVideo onMouseOver={this.handleEvent} ... /> |
onMouseOut |
function |
|
Function fired on onMouseOut event | <MDBCardVideo onMouseOut={this.handleEvent} ... /> |
onLoad |
function |
|
Function fired on onLoad event | <MDBCardVideo onLoad={this.handleEvent} ... /> |
sandbox |
boolean |
|
Adds optional sandbox values | <MDBCardVideo sandbox ... /> |
src |
string |
|
Required. The MDBCardVideo url | <MDBCardVideo src="https://www.youtu..." ... /> |
style |
object |
|
Add's additional styles. | <MDBCardVideo style={{color: "red"}} ... /> |
title |
string |
|
Defines component title | <MDBCardVideo titile="My title" ... /> |
ratio |
string |
|
Defines component ratio. Choose from "1by1", "4by3", "16by9" and "21by9" | <MDBCardVideo ratio="4by3" ... /> |
width |
number |
|
Defines component width | <MDBCardVideo width={300} ... /> |
API Reference: FlippingCard Properties
The table below shows the configuration options of the MDBFlippingCard component.
Name | Type | Default | Description | Example |
---|---|---|---|---|
className |
String |
|
Adds custom classes | <MDBFlippingCard className="customClass" /> |
flipped |
Boolean | false |
Flipps the Card initially | <MDBFlippingCard flipped /> |
innerTag |
String | div |
Changes the default html tag for component | <MDBFlippingCard innerTag="div" /> |
tag |
String | div |
Changes the default html tag for component's wrapper | <MDBFlippingCard tag="div" /> |