Manual installation (zip package)
Step 1
Download MDB 4 Angular Free or MDB 4 Angular Pro package
MDB Angular downloadStep 2
Unzip downloaded package and open it in the code editor
Step 3
Install dependencies. Depending on your node module manager, run:
npm install
yarn
Step 4
Run your application.
ng serve --o
Step 5
Explore our documentation (menu on the left). Choose components you like, copy it to your project and compose your website. And yes, it's that simple!
Note: Manual installation with a .zip package is the
easiest way, but many useful features are not available there.
To use the full potential of MDB and all available options, we recommend
installation through
MDB CLI.
MDB CLI
CLI installation is the most efficient way to use MDB. It enables options such as:
- Free hosting (supports custom domains, SSL, FTP access)
-
Install any MDB
package with a single command
- Easy updates with a single command
- Backend starter templates (Laravel, plain PHP, node.js & more)
- WordPress setup in 3 minutes (blog, ecommerce or blank project)
- Git repository for you and your team
Advanced installation (schemantics)
From MDB Angular 6.3.0 there's possibility to install MDB Angular Free by using ng add command.
Step 1
Create new project:
ng new your-project-name
Step 2
Add MDB Angular Free configuration:
ng add angular-bootstrap-md
NPM
Prerequisites
- Node.js: https://nodejs.org/en/
- Angular CLI:
npm install -g @angular/cli
- Git - required in npm installation of MDB Angular Pro package
Step 1
Create new angular project using Angular CLI command:
ng new your-angular-project --style=scss
Step 2
Open the project in the code editor
Step 3
Install @angular/cdk
package (required only for MDB Angular version 9.0.0 and later):
npm install @angular/cdk --save
Step 4
Install MDB Angular Free:
npm install angular-bootstrap-md --save
Step 5
Update the app.module.ts
file with the following code:
import { NgModule } from '@angular/core';
import { MDBBootstrapModule } from 'angular-bootstrap-md';
@NgModule({
imports: [
MDBBootstrapModule.forRoot()
]
});
Step 6
Update styles
and scripts
arrays in angular.json
file:
"styles": [
"node_modules/@fortawesome/fontawesome-free/scss/fontawesome.scss",
"node_modules/@fortawesome/fontawesome-free/scss/solid.scss",
"node_modules/@fortawesome/fontawesome-free/scss/regular.scss",
"node_modules/@fortawesome/fontawesome-free/scss/brands.scss",
"node_modules/angular-bootstrap-md/assets/scss/bootstrap/bootstrap.scss",
"node_modules/angular-bootstrap-md/assets/scss/mdb.scss",
"node_modules/animate.css/animate.css",
"src/styles.scss"
],
"scripts": [
"node_modules/chart.js/dist/Chart.js",
"node_modules/hammerjs/hammer.min.js"
],
Step 7
Install external libraries
npm install -–save chart.js@2.5.0 @types/chart.js @fortawesome/fontawesome-free hammerjs animate.css
Step 8
Run application
ng serve --o
Prerequisites
- Node.js: https://nodejs.org/en/
- Angular CLI:
npm install -g @angular/cli
- Git - required in npm installation of MDB Angular Pro package
Step 1
Create new angular project using Angular CLI command:
ng new your-angular-project --style=scss
Step 2
Open the project in the code editor
Step 3
Install @angular/cdk
package (required only for MDB Angular version 9.0.0 and later):
npm install @angular/cdk --save
Step 4
Generate new GitLab token (see token generation section)
Step 5
Use generated token to install MDB Angular Pro package
npm install git+https://oauth2:YOUR_TOKEN@git.mdbootstrap.com/mdb/angular/ng-uikit-pro-standard.git --save
Step 6
Update the app.module.ts
file with the following code:
import { MDBBootstrapModulesPro } from 'ng-uikit-pro-standard';
import { MDBSpinningPreloader } from 'ng-uikit-pro-standard';
import { NgModule } from '@angular/core';
...
imports: [
...
MDBBootstrapModulesPro.forRoot(),
...
],
providers: [
...
MDBSpinningPreloader,
...
]
Step 7
Update styles
and scripts
arrays in angular.json
file:
"styles": [
"node_modules/@fortawesome/fontawesome-free/scss/fontawesome.scss",
"node_modules/@fortawesome/fontawesome-free/scss/solid.scss",
"node_modules/@fortawesome/fontawesome-free/scss/regular.scss",
"node_modules/@fortawesome/fontawesome-free/scss/brands.scss",
"node_modules/ng-uikit-pro-standard/assets/scss/bootstrap/bootstrap.scss",
"node_modules/ng-uikit-pro-standard/assets/scss/mdb.scss",
"node_modules/animate.css/animate.css",
"src/styles.scss"
],
and scripts:
"scripts": [
"node_modules/chart.js/dist/Chart.js",
"node_modules/easy-pie-chart/dist/easypiechart.js",
"node_modules/screenfull/dist/screenfull.js",
"node_modules/hammerjs/hammer.min.js"
],
Step 8
Install external libraries
npm i --save chart.js@2.5.0 @types/chart.js easy-pie-chart@2.1.7 hammerjs@2.0.8 screenfull@3.3.0
@fortawesome/fontawesome-free animate.css
Step 9
If you want to use maps you will have to npm i --save @agm/core
and then add
import { AgmCoreModule } from '@agm/core';
to your app.module. To use them you will also need to
add it to your imports AgmCoreModule.forRoot({ apiKey: 'Your_api_key' })
Step 10
Run application
ng serve --o
Install specific version
When you install MDB from our GitLab server, the latest version of the library will be downloaded by default. You can add #version_number
at the end of the installation command in order to install specific MDB version.
npm install git+https://oauth2:YOUR_TOKEN@git.mdbootstrap.com/mdb/angular/ng-uikit-pro-standard.git#12.0.0 --save
Token generation
Step 1
Visit https://git.mdbootstrap.com and log in. If you are a PRO user, you should receive an email (from GitLab) with an activation link, after purchase (please check your spam folder as well). This email is send to the email address used for billing.
Step 2
From top right corner click at your avatar and choose "Setting → Access Tokens."
Step 3
Provide a Name for your token and choose "api" from scopes. Then click "Create personal access token"
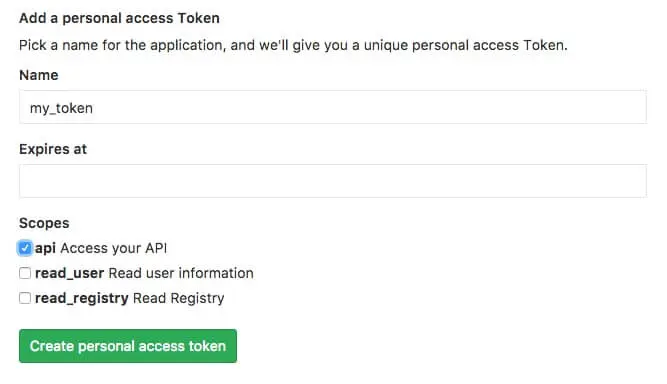
Step 4
Once your token will be generated make sure to copy it and store in safe place. You won't be able to access it again. In case of lose, you will have to generate new token again.

Snapshot builds (developer versions)
It's possible to download snapshot build (developer version).
MDB Angular Free:
git clone -b dev https://github.com/mdbootstrap/Angular-Bootstrap-with-Material-Design
MDB Angular Pro:
npm install git+https://oauth2:<your-auth-token>@git.mdbootstrap.com/mdb/angular/ng-uikit-pro-standard.git#dev --save
Please note that the developer version is the one we are working on when writing patches. This version is not stable and can cause problems in your project.
Plugin installation
Installation guide for the specific plugin is available in the plugin documentation.
List of all plugins can be found in the plugins and addons section.